Marshaling C++ Classes - P/Invoke with C#
Marshaling C++ classes for use in C# through Platform Invoke (P/Invoke) can be a complex yet powerful technique when integrating managed C# code with unmanaged C++ libraries.
Marshaling essentially involves translating data between managed and unmanaged memory spaces, allowing interoperability between the two environments.
In this tutorial, we'll explore the steps to marshal C++ classes to be consumed by C# code using P/Invoke.
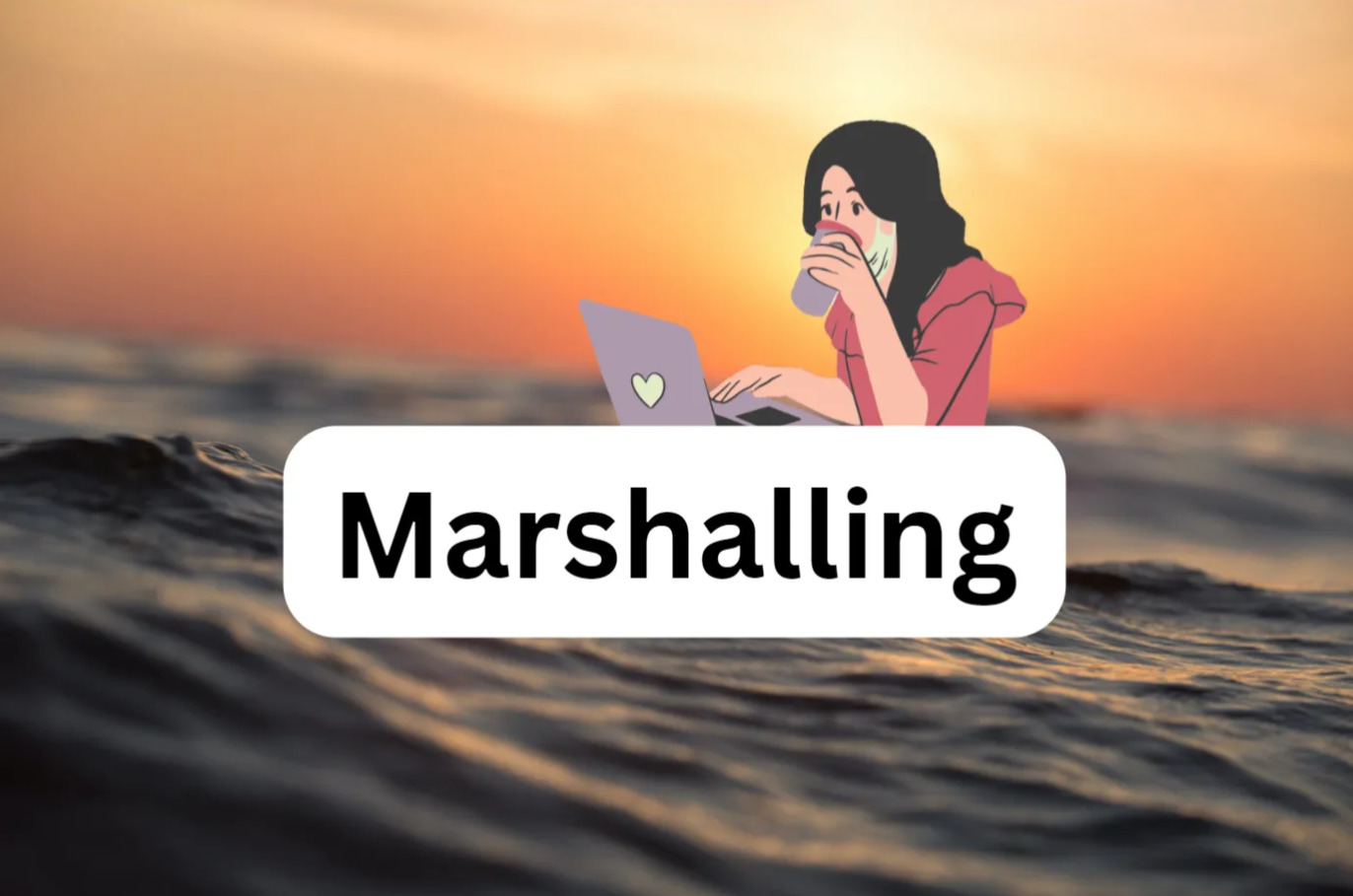
Prerequisites
To follow this tutorial, you should have:
- Basic knowledge of C++ and C# programming languages.
- Visual Studio or any other preferred IDE for C# development.
- Familiarity with P/Invoke and its concepts.
Understanding P/Invoke
Platform Invocation Services, commonly referred to as P/Invoke is a feature in .NET that allows managed code to call unmanaged functions implemented in dynamic link libraries (DLLs). It provides a way for managed code to interact with native code, enabling seamless integration between managed and unmanaged environments.
Marshaling C++ Classes
Marshaling C++ classes involves translating the structure and behavior of C++ classes into a format that can be understood and used by C#. This process requires careful consideration of data types, memory allocation, and function signatures.
Project Setup
Setting Up the C++ DLL Project:
- Open Visual Studio: Launch Visual Studio and select "Create a new project."
- Select Project Type: In the "Create a new project" window, choose "Visual C++" from the left panel, then select "Dynamic-Link Library (DLL)" from the available templates. Name your project (e.g., "ExampleLibrary") and choose a location to save it.
- Add Source Files: Right-click on the newly created project in the Solution Explorer, select "Add" > "New Item...". Choose "C++ File (.cpp)" and give it a meaningful name (e.g., "ExampleClass.cpp"). Add your C++ class implementation to this file.
- Add Header File: Similarly, add a header file for your C++ class. Right-click on the project, select "Add" > "New Item...", then choose "Header File (.h)" and provide a name (e.g., "ExampleClass.h"). Define your class interface in this header file.
- Build Configuration: Ensure that your project is configured to produce a DLL. Right-click on the project in Solution Explorer, choose "Properties," navigate to "Configuration Properties" > "General," and set "Configuration Type" to "Dynamic Library (.dll)".
- Build:Build your project to ensure there are no compilation errors.
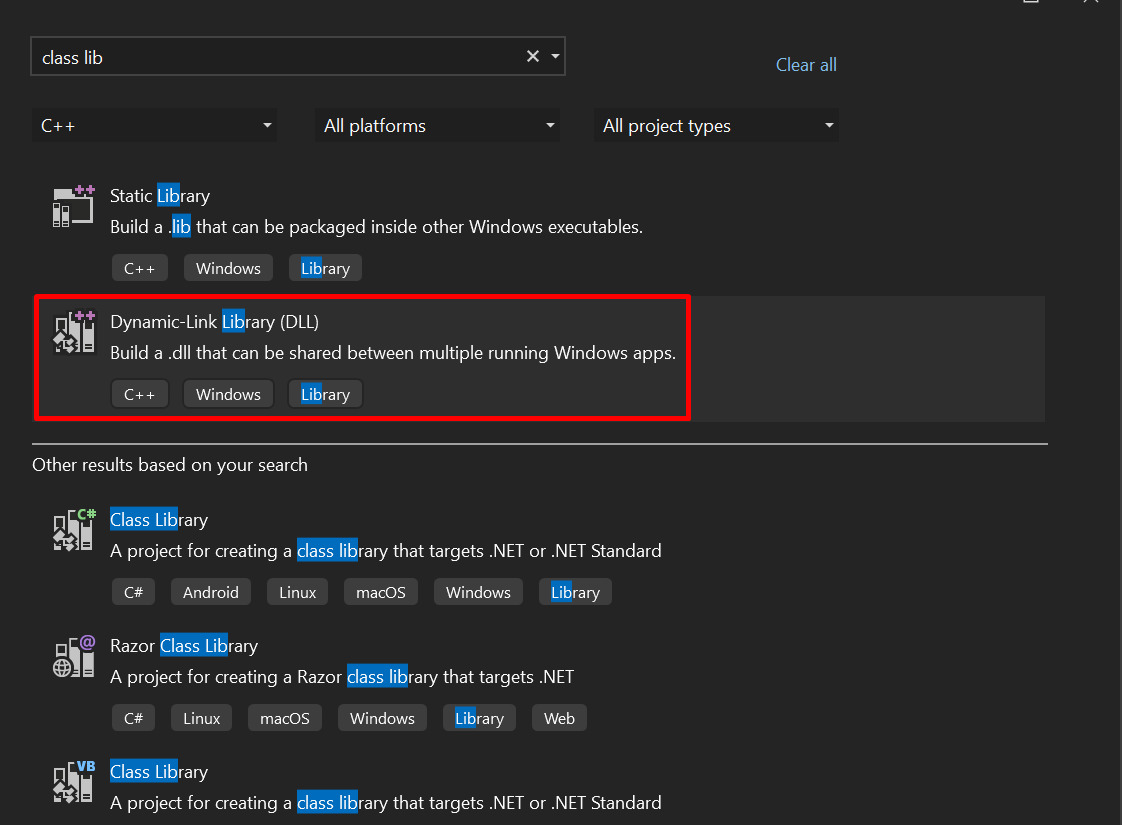
Setting Up the C# Console App:
- Open Visual Studio: Launch Visual Studio and select "Create a new project."
- Select Project Type: In the "Create a new project" window, choose "Visual C#" from the left panel, then select "Console App (.NET Core)" or ".NET Framework" from the available templates. Name your project (e.g., "ExampleApp") and choose a location to save it.
- Add Reference to C++ DLL: Right-click on the project in Solution Explorer, select "Add" > "Reference...". Browse to the location of your C++ DLL (built in the previous steps) and add it as a reference to your C# project.
- Build and Run: Build your C# project and ensure that it compiles without errors.

Pinvoke C++ Example
Let's consider a simple example where we have a C++ class representing a geometric point with x
and y
coordinates. We want
to create an instance of this class in C# and call its member functions from managed code.
Step 1: Define the C++ Class
Begin by creating or identifying the C++ class you want to use in your C# project. Ensure that the class has appropriate constructors, destructors, methods, and properties.
// C++ Class: Point.h
#pragma once
class Point {
public:
Point(int x, int y) : x(x), y(y) {}
int getX();
int getY();
private:
int x;
int y;
};
// C++ Class: Point.cpp
#include "pch.h"
#include "Point.h"
int Point::getX() {
return x;
}
int Point::getY() {
return y;
}
Step 2: Expose C++ Class to C#
To use the C++ class in C#, you need to create a C interface that exposes the functionalities of the C++ class.
// C++ Wrapper: PointWrapper.cpp
#include "pch.h"
#include "Point.h"
extern "C" __declspec(dllexport) Point * CreatePoint(int x, int y) {
return new Point(x, y);
}
extern "C" __declspec(dllexport) void DeletePoint(Point * point) {
// Check if the pointer is not null before deleting
if (point != nullptr)
{
delete point; // Properly delete the pointer
point = nullptr; // Set to null after deletion to avoid dangling pointer
}
}
extern "C" __declspec(dllexport) int GetX(Point * point) {
if (point != nullptr)
{
return point->getX();
}
}
extern "C" __declspec(dllexport) int GetY(Point * point) {
if (point != nullptr)
{
return point->getY();
}
}
Pinvoke C# Example
Now, we can create a C# program to consume this C++ class through P/Invoke.
Step 3: Define P/Invoke Signatures in C#
Now, in your C# project, declare the P/Invoke signatures to call the C++ functions.
// C# Point: Point.cs
using System.Runtime.InteropServices;
namespace CSharpApp
{
public class Point : IDisposable
{
public Pointer(int x, int y)
{
_pointer = CreatePoint(x, y);
}
// This finalizer will run when Garbage collection occurs, but
// only if the IDisposable.Dispose() method wasn't already called.
// It gives your base class the opportunity to finalize.
// Do not provide finalizer in types derived from this class.
~Pointer()
{
Dispose(disposing: false);
}
public void Dispose()
{
Dispose(disposing: true);
// This object will be cleaned up by the Dispose method.
// Therefore, you should call GC.SuppressFinalize to
// take this object off the finalization queue
// and prevent finalization code for this object
// from executing a second time.
GC.SuppressFinalize(this);
}
protected virtual void Dispose(bool disposing)
{
// Check to see if Dispose has already been called.
if (!_disposed)
{
// If disposing equals true, dispose all managed
// and unmanaged resources.
if (disposing)
{
// Dispose managed resources (C# objects).
}
// Call the appropriate methods to clean up
// unmanaged resources here.
// If disposing is false,
// only the following code is executed.
DeletePoint(_pointer);
_pointer = IntPtr.Zero;
// Note disposing has been done.
_disposed = true;
}
}
[DllImport("MarshalExample.dll")]
public static extern IntPtr CreatePoint(int x, int y);
[DllImport("MarshalExample.dll")]
public static extern void DeletePoint(IntPtr point);
[DllImport("MarshalExample.dll")]
public static extern int GetX(IntPtr point);
[DllImport("MarshalExample.dll")]
public static extern int GetY(IntPtr point);
public int Y
{
get { return GetX(_pointer); }
}
public int X
{
get { return GetY(_pointer); }
}
private IntPtr _pointer;
private bool _disposed = false;
}
}
Step 4: Use the C++ Class in C#
Now, you can utilize the C++ class in your C# code as follows:
// C# Program: Program.cs
namespace CSharpApp
{
internal class Program
{
static void Main(string[] args)
{
//var point = new Point(3, 4);
//Console.WriteLine($"X coordinate: {point.X}");
//Console.WriteLine($"Y coordinate: {point.Y}");
//point.Dispose();
using var point = new Point(3, 4);
Console.WriteLine($"X coordinate: {point.X}");
Console.WriteLine($"Y coordinate: {point.Y}");
}
}
}
Pinvoke .NET Core
If you're using .NET Core or .NET 5+, the process is the same, but you'll need to ensure compatibility with your target platforms.
Conclusion
Marshaling C++ classes for use in C# via P/Invoke provides a powerful mechanism for integrating native code with managed environments. However, it's essential to note the following:
- Ensure the C++ project is compiled with the correct architecture (x86/x64) to match your C# project.
- Be cautious with memory management; ensure proper cleanup of resources to prevent memory leaks.
- Handle exceptions gracefully, especially when dealing with unmanaged code.
- Understand the limitations and potential pitfalls of marshaling, especially concerning complex data types and memory layouts.